Running PHP from the Command Line - Basics
by Jarrod posted 2 years ago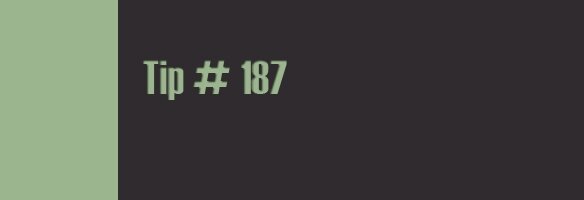
Here are a few of tips to share with you regarding running your PHP from the command line.
Running your PHP from the command line
That's as easy as calling "php" then your script name:
$ php script_name.php
Accepting arguments
You can pass arguments, also referred to as variables, to your script from the command line like so:
$ php script_name.php Jarrod
This example passes one argument, "Jarrod". To do something useful with this in PHP we use the array variable $argv. This is simply an array, created by PHP, that contains our arguments.
if (isset($argv[1])) {
echo 'My name is ' . $argv[1];
}
Notice we used the array index 1 and not 0? That is because $argv[0] is always the name that was used to run the script.
You can use multiple arguments, each argument will be a new array element.
$ php script_name.php Jarrod "cake, tea and ice cream"
Just to claify, the two arguments used above are:
- Jarrod
- cake, tea and ice cream
The second argument has spaces so I used quotes around the phrase.
if (isset($argv[1]) && isset($argv[2])) {
echo 'My name is ' . $argv[1] . ' and I like ' . $argv[2];
}
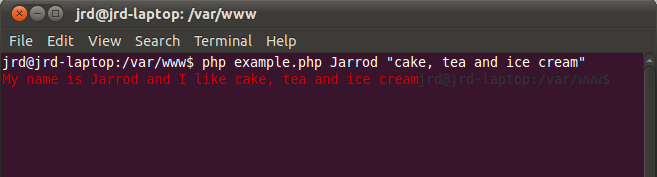
New Lines
Want to add a line break? <br /> won't work. Instead, you need to use the constant PHP_EOL.
if (isset($argv[1])) {
echo 'My name is ' . $argv[1] . PHP_EOL;
}
Colors
By prefixing a color code to your string you can change the text color. A color code looks like this for the color red: \033[1;31m (it's actually the last part that contains the color - in this example it's the 31 - but you still need the whole thing).
if (isset($argv[1])) {
echo "\033[0;31mMy name is " . $argv[1] . " and I like " . $argv[2] . "\033[0;30m";
}
A few points to make:
- There is no space between the color and my text. You can add a space - but the space will show up.
- At the end I include a color code. This is not like a closing tag, it's to reset the color back to black.
- I changed my single quotes to double quotes so the color code is actually parsed and not treated like a string!
Here are some common colors:
- Black = \033[0;30m
- Red = \033[0;31m
- Green = \033[0;32m
- Yellow = \033[0;33m
- Blue = \033[0;34m
- Purple = \033[0;35m
- Cyan = \033[0;36m
- White = \033[0;37m
Style
Similar to the color code - in fact it's the same thing, we just change a different part -you can set the style of the font; like bold, underline, etc.
This is done by changing the value shown in bold: \033[0;31m
A list of possible options are:
- 0 = normal
- 1 = bold
- 4 = underline
- 5 = blink
if (isset($argv[1])) {
echo "\033[5;31mMy name is " . $argv[1] . " and I like " . $argv[2] . "\033[0;30m";
}
There are no comments. Would you like to be the first?